Firebase Auth Plugin
This plugin allows you to log in though firebase auth for iOS(8+) and Android(4.0 +)
Functions:
firebaseAuth.init()
firebaseAuth.createAccount(email, password, listener)
--create account with emailemail(string)
password(string)
listener(function) event.isError(boolean value, true if successful and false if not) and event.error(string value, description of error, nil if isError is false)
firebaseAuth.signIn(email, password, listener)
--login with emailemail(string)
password(string)
listener(function) event.isError(boolean value, true if successful and false if not) and event.error(string value, description of error, nil if isError is false)
firebaseAuth.sendVerification(listener)
--send a verification email to logined in userlistener(function) event.isError(boolean value, true if successful and false if not) and event.error(string value, description of error, nil if isError is false)
firebaseAuth.resetPassword(email,listener)
--send an email to reset passwordemail(string) email to sent reset to
listener(function) event.isError(boolean value, true if successful and false if not) and event.error(string value, description of error, nil if isError is false)
firebaseAuth.isSignedIn()
returns boolean, true if logged in, false if notfirebaseAuth.isEmailVerified()
returns boolean, true if email on account logged in is verified, false if notfirebaseAuth.isAnonymous()
returns boolean, true if account is anonymous, false if notfirebaseAuth.getUID()
returns string, unique identifier for accountfirebaseAuth.getEmail()
returns string, email for accountfirebaseAuth.getDisplayName()
returns string, display name for accountfirebaseAuth.getPhoneNumber()
returns string, phone number for accountfirebaseAuth.getPhotoUrl()
returns string, url (http://) for account photofirebaseAuth.getRefreshToken(listener)
--get refresh token for accountlistener(function) event.isError(boolean value, true if successful and false if not), event.error(string value, description of error, nil if isError is false), and event.token (string value, refresh token)
firebaseAuth.setPhotoUrl(url,listener)
--set url for account photolistener(function) event.isError(boolean value, true if successful and false if not) and event.error(string value, description of error, nil if isError is false)
firebaseAuth.setEmail(email,listener)
--set email for accountlistener(function) event.isError(boolean value, true if successful and false if not) and event.error(string value, description of error, nil if isError is false)
firebaseAuth.setDisplayName(name,listener)
--set name for accountlistener(function) event.isError(boolean value, true if successful and false if not) and event.error(string value, description of error, nil if isError is false)
firebaseAuth.updatePassword(password,listener)
--update password for accountlistener(function) event.isError(boolean value, true if successful and false if not) and event.error(string value, description of error, nil if isError is false)
firebaseAuth.deleteAccount(listener)
--delete accountlistener(function) event.isError(boolean value, true if successful and false if not) and event.error(string value, description of error, nil if isError is false)
firebaseAuth.signOut(listener)
--sign out of accountlistener(function) event.isError(boolean value, true if successful and false if not) and event.error(string value, description of error, nil if isError is false)
firebaseAuth.sendVerificationCode(phoneNumber,listener)
--send verfication code to phone to be used to .signInWithPhoneNumberphoneNumber(string) phone number to send code to
listener(function) event.isError(boolean value, true if successful and false if not) and event.error(string value, description of error, nil if isError is false)
firebaseAuth.signInWithPhoneNumber(code,listener)
--send verfication code to phone to be used to .signInWithPhoneNumbercode(string) code sent with .sendVerificationCode
listener(function) event.isError(boolean value, true if successful and false if not) and event.error(string value, description of error, nil if isError is false)
firebaseAuth.signInWithApple(listener)
--sign in with applelistener(function) event.isError(boolean value, true if successful and false if not) and event.error(string value, description of error, nil if isError is false)
For iOS add provisioning profile support for apple sign in your apple devloper account, also add the following to your build.settings
For Android read the Firebase guide here
firebaseAuth.signInWithGoogle(idToken, accessToken,listener)
--sign in with googleidToken(string) id token for google
accessToken(string) access token for google
listener(function) event.isError(boolean value, true if successful and false if not) and event.error(string value, description of error, nil if isError is false)
firebaseAuth.signInWithFacebook(accessToken, listener)
--sign in with facebook(Please note you have to handle sign in yourself)accessToken(string) access token for facebook
listener(function) event.isError(boolean value, true if successful and false if not) and event.error(string value, description of error, nil if isError is false)
firebaseAuth.signInWithTwitter(token, secret, listener)
--sign in with twitter(Please note you have to handle sign in yourself)token(string)
secret(string)
listener(function) event.isError(boolean value, true if successful and false if not) and event.error(string value, description of error, nil if isError is false)
firebaseAuth.signInWithGithub(accessToken, listener)
--sign in with github(Please note you have to handle sign in yourself)accessToken(string)
listener(function) event.isError(boolean value, true if successful and false if not) and event.error(string value, description of error, nil if isError is false)
firebaseAuth.signInWithCustomToken(accessToken, listener)
--sign in with customer serveraccessToken(string)
listener(function) event.isError(boolean value, true if successful and false if not) and event.error(string value, description of error, nil if isError is false)
firebaseAuth.signInAnonymously(listener)
--sign in without account and used for securitylistener(function) event.isError(boolean value, true if successful and false if not) and event.error(string value, description of error, nil if isError is false)
firebaseAuth.isNetworkAvailable()
--check for wifi access, returns boolean, true if device has internet access, false if notfirebaseAuth.doesEmailExist(listener, email)
--checks if email is already registeredlistener(function) event.isError(boolean value, true if successful and false if not) and event.error(string value, description of error, nil if isError is false). If isError is false then event.doesEmailExist (boolean is returned, true if email exists, false if email does not exists).
email(string) email to check if registered
firebaseAuth.setAPNSToken(APNSToken, mode)
--set the apns tokenAPNSToken(string) Apple push notifications
mode(string) "prod"(production), "sandbox"(sandbox), and "unknown"
Make sure you download and include GoogleServices-Info.plist and put in root folder of corona project. Also add your google-services.json to your root folder and useGoogleServicesJson = true in build.settings. Please note according to firebase docs, you must have firebase cloud messaging enabled for phone login. Please follow setup on Corona FCM plugin page
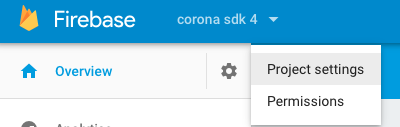

